Java – SpringMVC – 上传与下载
简介
使用SpringMVC实现上传与下载功能。
上传
浏览器向服务器上传文件数据,服务器接收文件数据。
下载
浏览器向服务器下载文件数据,服务器发送文件数据。
下载数据实现
@RequestMapping("/testDown")
public ResponseEntity<byte[]> testResponseEntity(HttpSession session) throws IOException {
//获取ServletContext对象
ServletContext servletContext = session.getServletContext();
//获取服务器中文件的真实路径
String realPath = servletContext.getRealPath("/static/img/1.jpg");
//创建输入流
InputStream is = new FileInputStream(realPath);
//创建字节数组
byte[] bytes = new byte[is.available()];
//将流读到字节数组中
is.read(bytes);
//创建HttpHeaders对象设置响应头信息
MultiValueMap<String, String> headers = new HttpHeaders();
//设置要下载方式以及下载文件的名字
headers.add("Content-Disposition", "attachment;filename=1.jpg");
//设置响应状态码
HttpStatus statusCode = HttpStatus.OK;
//创建ResponseEntity对象
ResponseEntity<byte[]> responseEntity = new ResponseEntity<>(bytes, headers,
statusCode);
//关闭输入流
is.close();
return responseEntity;
}
上传数据实现
@RequestMapping("/upload")
public String uploadFile(MultipartFile file, HttpSession httpSession) throws IOException {
// 取得上传目录
String uploadPath = httpSession.getServletContext().getRealPath("upload");
// 判断上传目录是否存在,如果不存在,就创建目录
File path = new File(uploadPath);
if (!path.exists()){
path.mkdirs();
}
// 取出上传文件的文件名
/**
* MultipartFile 是另一个第三方文件包,必须导入,否则 MultipartFile 为 Null
* MultipartFile 是一个接口,在导入到IoC Bean类时,需要导入它的实现类
* SpringMVC 对于Bean对象的调用,不是基于类型的,而是基于id
* 因此,配置 MultipartFile 的Bean类时,需要设置id值
*
* 关于导入第三方的包
* https://mvnrepository.com/artifact/commons-fileupload/commons-fileupload
* <dependency>
* <groupId>commons-fileupload</groupId>
* <artifactId>commons-fileupload</artifactId>
* <version>1.3.1</version>
* </dependency>
*
* 关于Bean对象的配置
* <bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver"></bean>
*/
String fileName = file.getOriginalFilename();
// 对上传路径进行合并(合并目录与文件名)
/**
* File.separator 是指按照操作系统的类型匹配对应的斜扛符
*/
String filePath = path + File.separator + fileName;
// 对上传的文件进行转存
file.transferTo(new File(filePath));
return "seccess";
}
关于防止上传文件重名的问题
对于文件重名的问题,OutputStream 默认会覆盖原文件的数据并写入新数据。
我们可以通过生成随机名称来避免重名问题。
UUID
是Java提供的一个随机生成编码名称的类,可以通过使用 UUID 生成的随机码对上传文件进行自定义名称。
改进后的代码:
@RequestMapping("/upload")
public String uploadFile(MultipartFile file, HttpSession httpSession) throws IOException {
// 取得上传目录
String uploadPath = httpSession.getServletContext().getRealPath("upload");
// 判断上传目录是否存在,如果不存在,就创建目录
File path = new File(uploadPath);
if (!path.exists()) {
path.mkdirs();
}
// 取出上传文件的文件名
/**
* MultipartFile 是另一个第三方文件包,必须导入,否则 MultipartFile 为 Null
* MultipartFile 是一个接口,在导入到IoC Bean类时,需要导入它的实现类
* SpringMVC 对于Bean对象的调用,不是基于类型的,而是基于id
* 因此,配置 MultipartFile 的Bean类时,需要设置id值
*
* 关于导入第三方的包
* https://mvnrepository.com/artifact/commons-fileupload/commons-fileupload
* <dependency>
* <groupId>commons-fileupload</groupId>
* <artifactId>commons-fileupload</artifactId>
* <version>1.3.1</version>
* </dependency>
*
* 关于Bean对象的配置
* <bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver"></bean>
*/
String fileName = file.getOriginalFilename();
/**
* 解决文件重名的问题
* 使用UUID生成随机码做文件名称
* 1.对文件名进行分割
* 2.取出文件名中的最后一个“.”出现的索引,直到字符串尾
* 3.加UUID码+字符串尾合成一个新的随机码名称
*/
String uuid = UUID.randomUUID().toString();
String fileType = fileName.substring(fileName.lastIndexOf(".")); // .jpg
fileName = uuid + fileType;
// 对上传路径进行合并(合并目录与文件名)
/**
* File.separator 是指按照操作系统的类型匹配对应的斜扛符
*/
String filePath = path + File.separator + fileName;
// 对上传的文件进行转存
file.transferTo(new File(filePath));
return "seccess";
}
THE END
0
二维码
打赏
海报
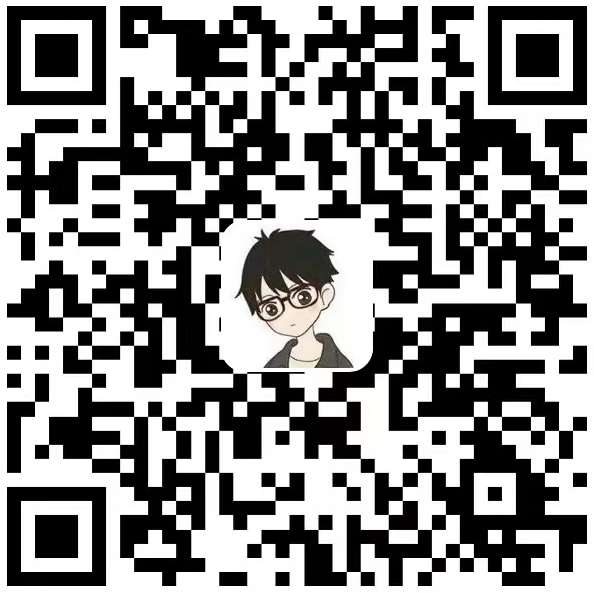
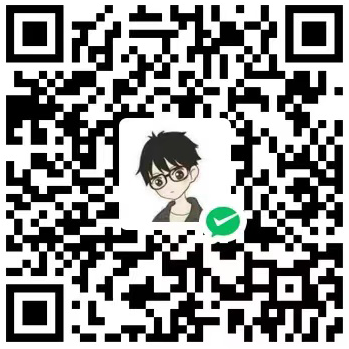
Java – SpringMVC – 上传与下载
简介
使用SpringMVC实现上传与下载功能。
上传
浏览器向服务器上传文件数据,服务器接收文件数据。
下载
浏览器向服务器下载文件数据,服务器发送文件数据。
下载数据实现
……
TZMing花园 - 软件分享与学习
共有 0 条评论